https://remix.ethereum.org/ with injected Web3 using Metamask is the simplest way to deploy smart contracts onto Eth-type blockchains. It requires no setup, aside from a Metamask installation. However, sometimes it just isn’t practical to use Remix.
Using Truffle to deploy smart contracts is another good option. Truffle allows you to deploy contracts via your local system directly to any network you choose.
Create a New Repo and Install Truffle
mkdir myproject
cd myproject
npm install truffle
npm install @truffle/hdwallet-provider
truffle init
This will initialize a new truffle project in the myproject folder. It will also install a wallet provider, which will allow you to make use of your existing eth wallet by providing the secret mnemonic phrase.
Create a New Solidity File
Add the following code to the contracts folder of your project in a file named ShowOwner.sol:
pragma solidity >=0.8.1;
contract ShowOwner{
address private owner;
constructor(){
owner = msg.sender;
}
function getOwner() public view returns(address){
return owner;
}
}
Upon creation the contract records the address of the contract creator (your wallet address). Calling the getOwner() function will return the address of the contract creator.
Contract Deployment
Enter the migrations folder and create this file: 2_deploy_contracts.js
The contents of 2_deploy_contracts.js should be:
var ShowOwner = artifacts.require("ShowOwner");
module.exports = function(deployer) {
deployer.deploy(ShowOwner);
// Additional contracts can be deployed here
};
This code simply tells truffle to register the ShowOwner contract and to deploy.
Get Rid of Extraneous Migrations
Truffle has a feature which automatically registers new migrations to the blockchain. This means that every time you deploy a contract in a Truffle project, the record of that change is saved as a SECOND migration contract on chain. Some may find this useful, but for most this just serves as an unnecessary extra expense. After all, every time we deploy a contract to an eth blockchain it costs money in the form of gas.
You can disable this by changing the 1_initial_migration.js file in the migrations folder to:
const Migrations = artifacts.require("Migrations");
module.exports = function (deployer) {
if(deployer.network_id != 97){
deployer.deploy(Migrations);
}
};
This makes it so that migration recording will not take place if the network id is 97. We’ll talk about network ids in the next section.
Configure the Network
We’ll be deploying our contract to the Binance Smart Chain Testnet. In the root folder of your project, update truffle-config.js to:
var HDWalletProvider = require("truffle-hdwallet-provider");
const MNEMONIC = 'YOUR_MNEMONIC';
module.exports = {
networks: {
bsctestnet: {
provider: function() {
return new HDWalletProvider(MNEMONIC, "https://data-seed-prebsc-2-s3.binance.org:8545")
},
network_id: 97,
gas: 4000000
}
},
// Set default mocha options here, use special reporters etc.
mocha: {
// timeout: 100000
},
// Configure your compilers
compilers: {
solc: {
version: "0.8.1"
}
},
db: {
enabled: false
}
};
Make sure to replace ‘YOUR_MNEMONIC’ with the 12 word mnemonic from your wallet. If you are using git for version control, make sure you add truffle-config.js to your .gitignore file, otherwise you may end up sharing your private mnemonic with the world! You WILL lose all of the cypto in your wallet if you do that.
Note: Metamask allows you to create multiple accounts for a single wallet mnemonic. Truffle will make use of Account 1 by default.
Here we’ve created a network called bsctestnet. The BSC testnet node is at https://data-seed-prebsc-2-s3.binance.org:8545. Remember the network id we’d mentioned in our migration omission script earlier? It shows up here. The network is is 97.
You might have noticed the compilers section. This allows you to set your desired solc compiler version. The default is version 0.5.4. In our case, compiler version 0.5.4 won’t work because our contract is set to use version 0.8.1 or higher.
Contract Deployment
Deploy the contract by running the following command in the root folder of the project:
truffle deploy --network bsctestnet
You should see something similar to the following:
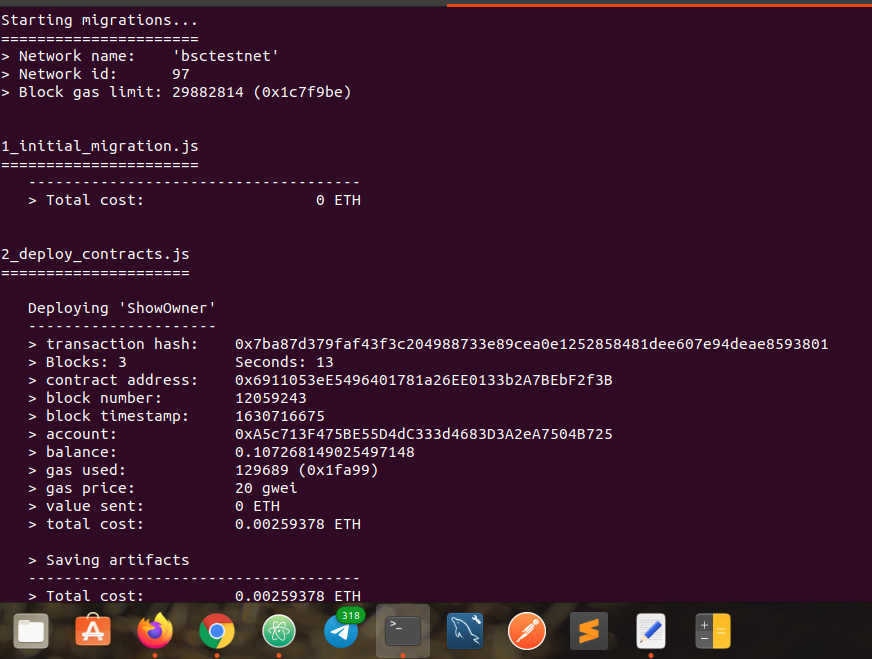
You can view the contract we’ve just deployed on the BSC Testnet Explorer here:
https://testnet.bscscan.com/address/0x6911053eE5496401781a26EE0133b2A7BEbF2f3B
The following link lets you view the read functions of the contract:
https://testnet.bscscan.com/address/0x6911053eE5496401781a26EE0133b2A7BEbF2f3B#readContract
Congratulations! You’ve deployed your contract successfully using Truffle!